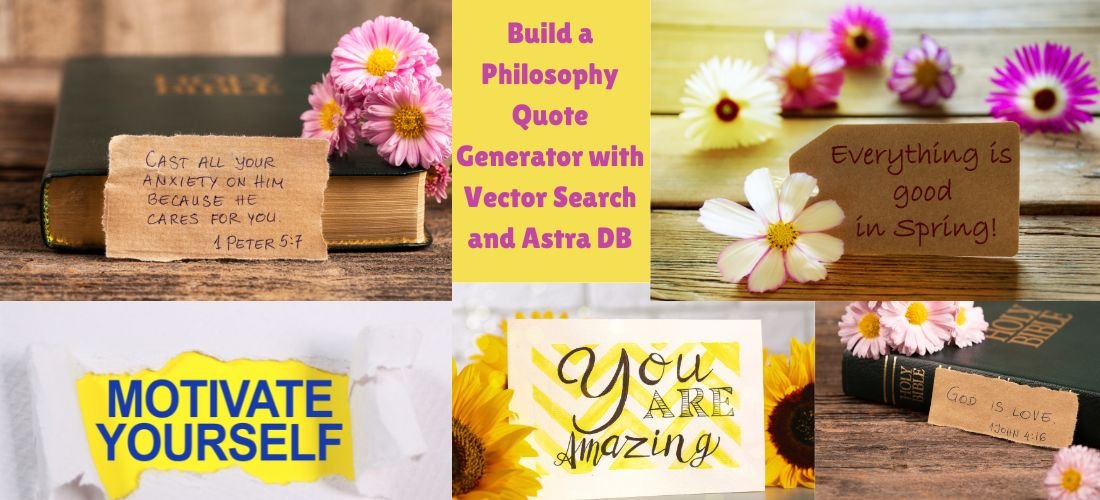
Build a Philosophy Quote Generator with Vector Search and Astra DB (part 3)
In this final part of our series on building a philosophy quote generator, we will integrate vector search with Astra DB and deploy our application. By the end of this tutorial, you’ll have a fully functional quote generator capable of returning contextually relevant philosophy quotes based on user input. This article assumes you’ve already set up your Astra DB instance, populated it with quotes, and generated embeddings for those quotes.
Build a Philosophy Quote Generator with Vector Search and Astra DB (part 3): Step-by Step
Step 1: Implementing Vector Search
Vector search is a powerful technique that allows us to find quotes that are semantically similar to a user’s query. Instead of relying on keyword matching, vector search uses the meanings behind words, enabling more accurate and relevant results.
1.1 Generating Query Embeddings
The first step in vector search is to convert the user’s query into a vector representation. We use the same pre-trained model (like Sentence-BERT) that we used to generate embeddings for the quotes. This ensures that both the quotes and the query exist in the same vector space.
Copy this Code
# Load the pre-trained model
model = SentenceTransformer(‘all-MiniLM-L6-v2’)
# Example query
query = “What is the meaning of life?”
# Generate the embedding for the query
query_embedding = model.encode(query)
1.2 Performing Vector Search in Astra DB
With the query embedding ready, the next step is to search for the most similar quote embeddings in Astra DB. Astra DB’s vector search functionality allows us to find the closest matches efficiently.
To perform vector search, we calculate the similarity between the query vector and each quote vector stored in the database. Cosine similarity is commonly used for this purpose, but Euclidean distance is another option. Here’s how you might implement this using Astra DB’s capabilities:
Copy this Code
# Connect to Astra DB
cluster = Cluster([‘your_astra_db_host’], port=29042)
session = cluster.connect(‘your_keyspace’)
# Execute the vector search query
query = “””
SELECT id, quote_text, author, embedding_vector
FROM quotes_table
“””
rows = session.execute(query)
# Function to calculate cosine similarity
def cosine_similarity(a, b):
return np.dot(a, b) / (np.linalg.norm(a) * np.linalg.norm(b))
# Find the most similar quotes
similarity_scores = []
for row in rows:
quote_vector = np.array(row.embedding_vector)
similarity = cosine_similarity(query_embedding, quote_vector)
similarity_scores.append((row.id, row.quote_text, row.author, similarity))
# Sort by similarity
similarity_scores.sort(key=lambda x: x[3], reverse=True)
# Get top N results
top_results = similarity_scores[:5]
Step 2: Optimizing the Search Results
After retrieving the closest matches, we can further optimize the search results to ensure they are as relevant as possible.
2.1 Adjusting Vector Search Parameters
Fine-tuning the parameters of your vector search can greatly impact the relevance of the returned quotes. For instance, adjusting the number of nearest neighbors (k-NN) allows you to control how many quotes are retrieved. You may also apply additional filtering based on the philosophical themes or the specific authors you want to prioritize.
2.2 Ranking and Relevance
While vector similarity provides a good starting point, you can further refine the results by implementing custom ranking mechanisms. For example, you might prioritize quotes from certain philosophers or specific philosophical eras based on the context of the user query. Combining vector similarity with these additional filters can yield highly relevant results.
Step 3: Deploying the Application
With the backend ready, the next step is to package and deploy the application. Here’s how you can do it:
3.1 Backend Deployment
The backend of the application, which handles the vector search and database interactions, can be deployed as a microservice. You can use popular frameworks like Flask or FastAPI to create a RESTful API that interacts with Astra DB and handles user queries.
Copy this Code
app = Flask(__name__)
@app.route(‘/search’, methods=[‘POST’])
def search():
query = request.json.get(‘query’)
# Generate query embedding and perform vector search
# (Similar to the previous steps)
results = perform_vector_search(query)
return jsonify(results)
if __name__ == ‘__main__’:
app.run(host=’0.0.0.0′, port=5000)
3.2 Frontend Integration
For the frontend, create a simple user interface where users can input their queries and view the results. You can use HTML, CSS, and JavaScript for a basic web app or a framework like React for a more dynamic experience. The frontend sends user queries to the backend API and displays the returned quotes.
3.3 Cloud Deployment
Deploy the entire application on a cloud platform like AWS, Google Cloud, or Azure. Make sure to:
- Containerize the Application: Use Docker to containerize your backend service, ensuring it can run consistently across different environments.
- Set Up Continuous Integration/Continuous Deployment (CI/CD): Implement a CI/CD pipeline to automate the deployment process and make updates seamless.
- Monitor Performance: Use monitoring tools to track the application’s performance, particularly focusing on the latency of vector searches and database queries.
Step 4: Testing and Refinement
4.1 User Testing
Thoroughly test the application with a variety of philosophical queries to ensure it returns meaningful and accurate quotes. Pay attention to edge cases, such as ambiguous or highly abstract queries, to see how well the system handles them.
4.2 Iteration Based on Feedback
Gather feedback from users and make improvements to both the vector search algorithm and the user interface. This could involve adjusting the search parameters, improving the UI/UX, or expanding the database of quotes.
Conclusion
In this three-part series, we successfully built a philosophy quote generator using Astra DB and vector search. We designed a database schema, created a table for storing quotes, and indexed the vector column to enable efficient similarity searches. By leveraging vector search, our quote generator can find quotes with similar meanings and themes, allowing users to explore the vast world of philosophy in a unique and engaging way. With this powerful tool, philosophy enthusiasts can discover new quotes, authors, and ideas, and gain a deeper understanding of the subject. The possibilities for expansion and improvement are endless, and we hope this project inspires others to explore the intersection of technology and philosophy.
Read Also: 139.59.223.45 IP Address Information | AI Reels AiperezTechCrunch